var, let, and const in JavaScript: What’s the Difference?
If you’ve just started learning JavaScript, you’ve probably been seeing three different keywords being used to declare variables: var
, let
, and const
. Each of these keywords has its own behavior and use cases.
This article will explore those differences.
The var
keyword
The var
keyword was originally used for declaring variables in JavaScript. If you’ve browsed an old codebase or an older JavaScript tutorial, you have probably seen it.
var
has been around since JavaScript’s inception and is still widely used (surprisingly).
It has some quirks that can lead to unexpected behavior.
For example, variables declared with var
are function-scoped, meaning that they are accessible within the entire function that they are declared in. This can lead to issues with variable hoisting, where variables are declared at the top of their scope regardless of where they are actually declared in the code. This can lead to bugs and make it harder to understand code.
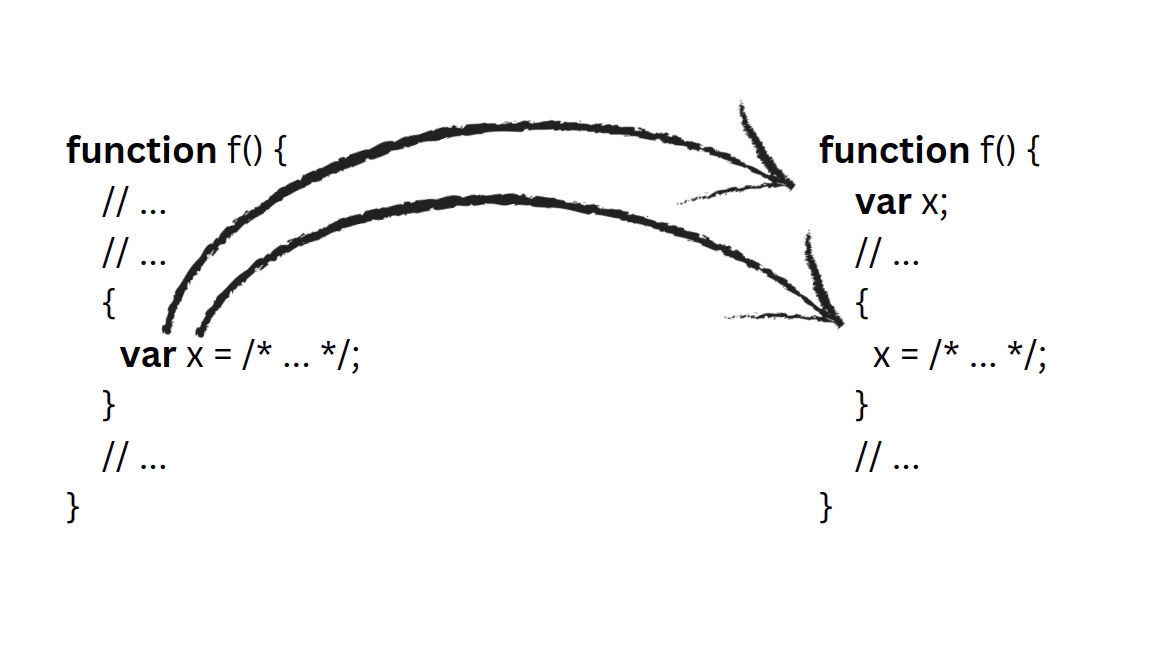
Example of variable hoisting
The let
keyword
let
was introduced in ES6 as a replacement for var
.
let
is block-scoped, meaning that variables declared with let are only accessible within the block that they are declared in. This makes it easier to reason about code and prevents variable hoisting issues. Additionally, variables declared with let can be reassigned later in the code.
This can be useful in cases where you need to reassign a variable within a loop or conditional statement.
The const
keyword
const
is similar to let
in that it is also block-scoped. However, variables declared with const
cannot be reassigned. This makes const
useful for declaring values that should not change throughout the course of the program. It also helps prevent accidental variable reassignment, which can lead to bugs and lots of headaches.
In general, it is best to use let
or const
instead of var
when declaring variables in JavaScript. let
should be used for variables that may be reassigned, while const
should be used for variables that should not be reassigned.
Using var
, let
, and const
Let’s take a look at an example of using these three keywords.
// Using var
function example() {
var x = 1;
if (true) {
var x = 2;
console.log(x); // Output: 2
}
console.log(x); // Output: 2
}
// Using let and const
function example() {
const x = 1;
if (true) {
let x = 2;
console.log(x); // Output: 2
}
console.log(x); // Output: 1
}
In the first code block, using var
leads to unexpected behavior because the variable x
is hoisted to the top of the function and is therefore accessible within the entire function.
This means that the value of x
is changed within the if statement and then again outside of it. In the second code block, using let
and const
ensures that the variable x
is only accessible within its block, making it easier to reason about the code.
Another important point to consider when using let
and const
is that they are not subject to variable hoisting, such as var
. This means that variables declared with let
or const
are only accessible after they have been declared. This can prevent bugs that can occur when variables are accessed before they have been declared.
Conclusion
var
, let
, and const
are all used for declaring variables in JavaScript, but they have different scoping, hoisting, and reassignment behaviors. In general, it is recommended to use let
or const
instead of var
when declaring variables, depending on whether they need to be reassigned or not. It is also important to consider the scoping and hoisting behavior of let
and const
, as well as their use in loops and the global scope, when writing JavaScript code.
Recommended Resource
Want to learn Advanced JavaScript?
Check out JavaScript: The Advanced Concepts, taught by an amazing instructor, Andrei Neagoi.
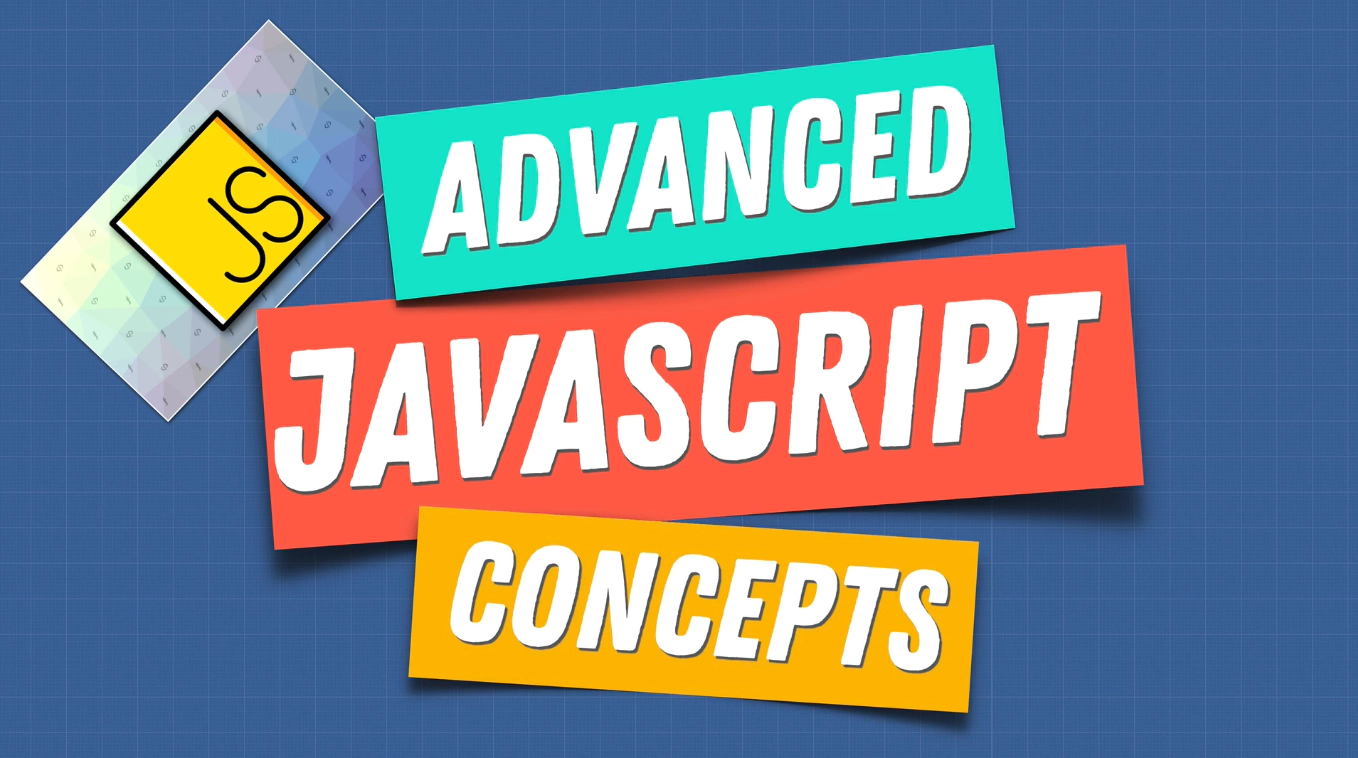
In this course, you will learn:
- Advanced JavaScript Practices.
- Scope and Execution Context.
- Object Oriented Programming & Functional Programming.
- Asynchronous JavaScript + Event Loop.
And much more!
Get 10% off by using code FRIENDS10.
Disclosure: We are affiliated with ZTM (Zero to Mastery), so if you happen to be interested in the course above and purchase it through our link, we will recieve a small commission of the sale (at no extra cost to you). Please also note that we have browsed ZTM courses and have found them to be some of the best material on the web. We don’t recommend them solely for personal gain.
Thank you for the support!
Related Posts
The Art of Data Visualization: Exploring D3.js
Data is everywhere, flowing into our applications from various sources at an unprecedented rate. However, raw data alone holds little value unless it can be transformed into meaningful insights.
Read moreJavaScript’s Secret Weapon: Supercharge Your Web Apps with Web Workers
During an interview, I was asked how we could make JavaScript multi-threaded. I was stumped, and admitted I didn’t know… JavaScript is a single-threaded language.
Read moreCreating a NodeJS Budgeting Tool
In this article, we’re going to show you how to read and write to a .txt file using NodeJS by creating a small budgeting CLI (Command Line Interface).
Read more