Three JavaScript Projects You Should Know About
If you’ve been paying attention to JavaScript these last few years, you would have noticed the high-number of frameworks, libraries, and tools being released. It seems there’s something new released every single day. Some call it framework hell.
Whether or not you’ve been paying attention to all of this, here are three very interesting projects that may spark your interest.
Mermaid
The first on this list is Mermaid, “a JavaScript-based diagramming and charting tool that uses Markdown-inspired text definitions and a renderer to create and modify complex diagrams.” It is an incredible tool that would be useful to every developer that writes any sort of documentation.
Fun fact: Mermaid was nominated and won the JS Open Source Awards (2019) in the category “The most exciting use of technology.”
If you are familiar with Markdown you should have no problem learning Mermaid’s Syntax.
Mermaid pie chart example:
This pie chart is accomplished with the following markdown:
pie
"Dogs" : 386
"Cats" : 85.9
"Rats" : 15
Qwik
Next up on our list is Qwik, a “new approach to performance optimization.” Qwik brings in an entirely new rendering paradigm called resumability, which means that Qwik “serializes the application’s state and framework state into HTML upon rendering the application.” This means your application’s PageSpeed score should be near perfect no matter how big and complex your JavaScript code is.
Another unique feature of Qwik is the ability to lazy load the code. This means that JavaScript will never load, nor execute until it is needed. For example, if you have an HTML <button>
with an onclick
handler, Qwik will only execute the code from the onclick
handler when the button is clicked, thus “the amount of code downloaded to the client is proportional to the complexity of the user interaction, not the size of all components on the current route.” If you’re already a React developer, then you already know how to build Qwik applications, as it’s built on top of JSX, functional components, and reactivity.
Take a look at the example from the Qwik website:
import { component$, useStore } from '@builder.io/qwik';
export const MyCmp = component$((props) => {
const state = useStore({ count: 0 });
return (
<>
<span>
Hello, {props.name}: {state.count}
</span>
<button onClick$={() => {state.count++ }}>
Increment
</button>
</>
);
});
It looks very familiar, no?
If you want to learn more about Qwik, you might find this presentation from Misko Hevery (creator of Angular and AngularJS) helpful: How to Remove 99% of JavaScript from the Main Thread.
Bun
As of writing this article, Bun is a new JavaScript runtime with a native bundler, transpiler, task runner, and npm client built-in. The goal of Bun “is to run most of the world’s JavaScript outside of browsers, bringing performance and complexity enhancements to your future infrastructure, as well as developer productivity through better, simpler tooling.” Rather than using V8, Bun uses the JavaScriptCore engine, which performs a little faster than V8. Bun is also written in the Zig programming language.
According to the website, Bun was built from scratch to focus on three main things:
- Start fast (it has the edge in mind).
- New levels of performance (extending JavaScriptCore, the engine).
- Being a great and complete tool (bundler, transpiler, package manager).
On the Bun website, we see the following graph showing Bun to be significantly faster than Node and Deno:
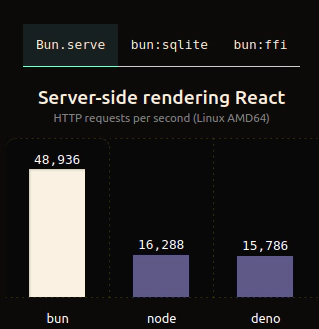
Aside from the speed, Bun is very exciting because of what it offers to simplify your life as a developer. Check it out:
- Web APIs like
fetch
,WebSocket
, andReadableStream
are built-in node_modules
: bun implements Node.js' module resolution algorithm, so you can use npm packages in Bun. ESM and CommonJS are supported, but Bun internally uses ESM- In Bun, every file is transpiled. TypeScript & JSX just work
node:fs
,node:path
: Bun natively supports a growing list of Node.js core modules along with globals like Buffer and process
Do keep in mind that Bun is experimental software. Use at your own risk!
Conclusion
There we have it, three neat JavaScript projects you should be aware of. Do you have a favorite JavaScript project? Let us know in the comments below, we’ll be happy to check it out!
Related Posts
The Best Way to Learn How to Code in 2024
Codecademy has been around for awhile now, but we felt this masterful site deserved to be highlighted in an article. It is one of the resources I originally used when I was learning how to code.
Read moreUnveiling the Fascination of the Collatz Conjecture: Exploring Sequence Creation with JavaScript
The Collatz Conjecture, also known as the 3x+1 problem, is a fascinating mathematical puzzle that has intrigued mathematicians for decades. It has sparked publications with titles such as The Simplest Math Problem Could Be Unsolvable, or The Simple Math Problem We Still Can’t Solve because it is, indeed, rather simple-looking.
Read moreThe Art of Data Visualization: Exploring D3.js
Data is everywhere, flowing into our applications from various sources at an unprecedented rate. However, raw data alone holds little value unless it can be transformed into meaningful insights.
Read more