A Deep Dive into Recursion with Examples in JavaScript
Recursion is a programming technique that essentially allows a function to call itself repeatedly until a specific condition is met.
Recursion can be used to solve complex problems by breaking them down into smaller, more manageable sub-problems. It can be used to simplify complex algorithms and improve code readability. It’s a common theme in developer interviews – you should know it well.
In this article, we’ll explore recursion using JavaScript and provide examples to illustrate its usage.
What is recursion?
Recursion is a programming technique where a function calls itself repeatedly until a base condition is met. The base condition is a condition that, once met, will stop the function from calling itself.
Recursion is similar to a loop, but it uses function calls instead of iterations. Recursion can be a powerful technique for solving complex problems, as it allows you to break down a problem into smaller, more manageable sub-problems.
If you’d like a little laugh, do a quick Google search for “recursion”, and you’ll be greeted with a fun joke:
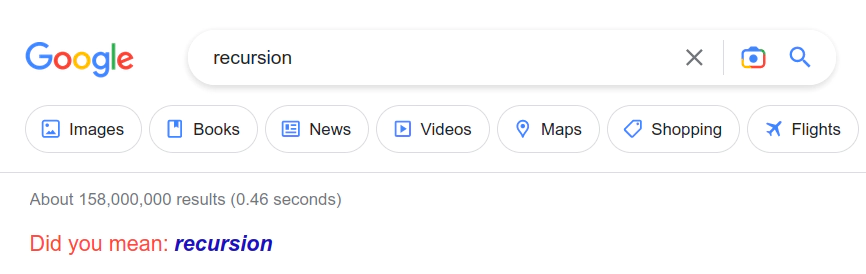
Recursion in JavaScript
JavaScript supports recursion just like any other programming language. Here is an example of a recursive function in JavaScript that calculates the factorial of a number:
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5)); // Output: 120
Here, the factorial()
function calls itself until it reaches the base condition, which is n === 0
. The function calculates the factorial by multiplying the current number n
with the factorial of n-1
. Once n
reaches 0
, the function stops calling itself and returns 1
.
Another example of a recursive function in JavaScript is the Fibonacci sequence:
function fibonacci(n) {
if (n === 0 || n === 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
console.log(fibonacci(6)); // Output: 8
The fibonacci()
function calls itself recursively to calculate the nth number in the Fibonacci sequence. The base condition is when n
is 0
or 1
, at which point the function returns n
. Otherwise, the function calculates the nth number by adding the previous two numbers in the sequence, which are calculated recursively using the fibonacci()
function.
Recursion vs. Iteration
Recursion and iteration are both techniques for solving problems that involve repeating a process multiple times.
However, there are some key differences between the two. Recursion involves a function calling itself, while iteration uses loops to repeat a process.
Recursion can be more concise and easier to read in some cases, but it can also be less efficient and more prone to stack overflow errors.
Consider the following example of calculating the sum of an array using recursion and iteration:
// Using recursion
function sum(arr) {
if (arr.length === 0) {
return 0;
} else {
return arr[0] + sum(arr.slice(1));
}
}
console.log(sum([1, 2, 3, 4, 5])); // Output: 15
// Using iteration
function sum(arr) {
let result = 0;
for (let i = 0; i < arr.length; i++) {
result += arr[i];
}
return result;
}
console.log(sum([1, 2, 3, 4, 5])); // Output: 15
As we can see, both recursion and iteration can be used to calculate the sum of an array. The recursive function sum()
calls itself with a smaller version of the array until it reaches the base condition where the array length is 0
.
The iterative function sum()
uses a loop to add up all the elements in the array and returns the total sum. In this case, the iterative function may be more efficient than the recursive function, as recursion can lead to stack overflow errors when the function is called too many times.
Common pitfalls of recursion
While recursion can be a powerful technique for solving complex problems, there are some common pitfalls to be aware of:
- Stack overflow: Recursion can cause stack overflow errors when the function is called too many times. This can happen when the base condition is not defined correctly or when the recursion depth is too high.
- Performance: Recursion can be less efficient than iteration, especially for large input sizes. Each recursive call creates a new stack frame, which can add up to a significant amount of memory usage.
- Debugging: Recursive functions can be more difficult to debug than iterative functions, as the function call stack can be deeper and more complex.
Conclusion
Recursion is a powerful programming technique that allows a function to call itself repeatedly until a base condition is met. It can be used to solve complex problems by breaking them down into smaller, more manageable problems.
We hope this article was useful to you. Drop a reaction and/or comment below!
FAQ
Q: What is recursion?
A: Recursion is a technique in programming where a function calls itself to solve a problem.
Q: What are the advantages of using recursion?
A: Recursion can simplify code, make it easier to read and understand, and can be a more elegant solution to certain problems.
Q: What are the disadvantages of using recursion?
A: Recursion can be less efficient than iterative solutions, can lead to stack overflow errors if not implemented correctly, and can be harder to debug and understand for some programmers.
Q: What is the base case in recursion?
A: The base case is the condition in a recursive function that stops the recursion from continuing. It is typically the simplest version of the problem that can be solved without recursion.
Q: What is the recursive case in recursion?
A: The recursive case is the condition in a recursive function that calls itself to solve a more complex version of the problem.
Q: What is the difference between direct and indirect recursion?
A: Direct recursion is when a function calls itself directly, while indirect recursion is when a function calls another function, which then calls the original function.
Q: What is tail recursion?
A: Tail recursion is a form of recursion where the recursive call is the last operation in the function, allowing the compiler to optimize the code by not using additional stack space.
Q: How can you avoid stack overflow errors in recursion?
A: You can avoid stack overflow errors in recursion by ensuring that the recursion has a base case, limiting the depth of the recursion, and using tail recursion when possible.
Q: What are some common examples of problems that can be solved using recursion?
A: Some common examples of problems that can be solved using recursion include factorial calculation, Fibonacci sequence generation, tree traversal, and binary search.
Related Posts
The Art of Data Visualization: Exploring D3.js
Data is everywhere, flowing into our applications from various sources at an unprecedented rate. However, raw data alone holds little value unless it can be transformed into meaningful insights.
Read moreJavaScript’s Secret Weapon: Supercharge Your Web Apps with Web Workers
During an interview, I was asked how we could make JavaScript multi-threaded. I was stumped, and admitted I didn’t know… JavaScript is a single-threaded language.
Read moreCreating a NodeJS Budgeting Tool
In this article, we’re going to show you how to read and write to a .txt file using NodeJS by creating a small budgeting CLI (Command Line Interface).
Read more